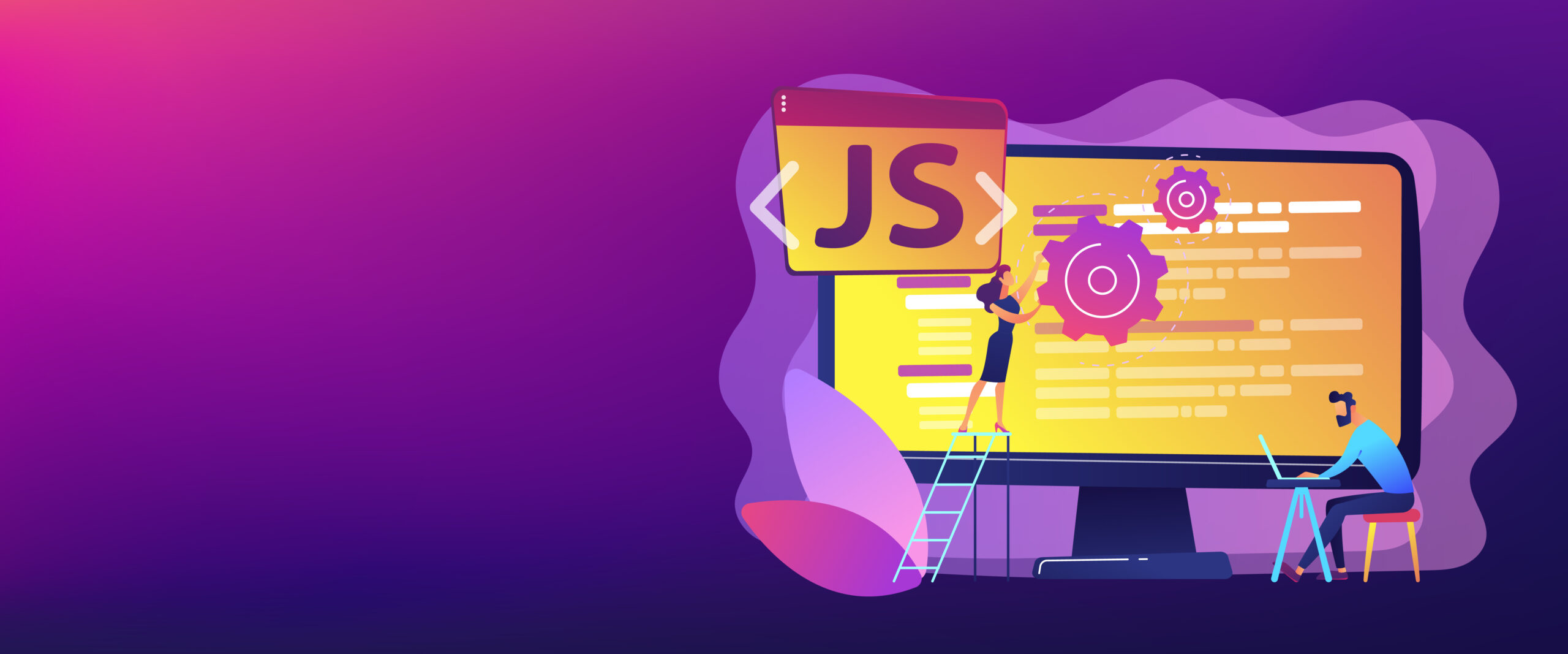
This article explores JavaScript Autoboxing and is part of our Byte Sized series.
Let’s start by looking at this simple code snippet
const myString = "Hello World";
console.log(myString.toUpperCase()); // prints HELLO WORLD
console.log(myString.length); // prints 11
We know that a string in JavaScript is a primitive type (that is, it has no methods or properties), but here is looks like that the variable myString is an object with a method toUpperCase() and a property called length.
So what’s the deal here?
Due to a feature known as “autoboxing”, JavaScript primitives appear to have methods and properties. What actually happens is that a wrapper object associated with the primitive is automatically accessed instead. Except for null and undefined, all primitive values have object equivalents that wrap around the primitive values:
- String for the string primitive.
- Number for the number primitive.
- BigInt for the bigint primitive.
- Boolean for the boolean primitive.
- Symbol for the symbol primitive.
The wrapper’s valueOf() method returns the primitive value.
A few things to remember here (if you’re new to JavaScript or brushing up your skills):
- Primitives in JavaScript are immutable. A primitive variable can be replaced but cannot be directly altered.
- Non-Primitive data types (or more accurately called Reference Types) are things likes Arrays, Objects.
- A primitive data type cannot be assigned a null value. This is not very clear at first, but abundantly clear if you use TypeScript.
To elaborate the last point, let’s looks at the code snippet here:
let myString = null;
console.log(typeof myString); // object
myString = "Apple";
console.log(typeof myString); // string
console.log(myString.length);
/* The line above is valid if myString is assigned a value like "Apple"
If not, it will result in a runtime exception.
*/
The above are some of the perils of implicit type conversion in JavaScript and can lead to unexpected behaviour. Therefore, we recommend using TypeScript.
Tip: For more posts like this, please visit our Software Engineering blog! We try to add posts like this regularly as part of our constant learning and upgrade initiatives.